Explaining Object-Oriented Programming in Python: A Detailed Guide
8/20/20247 min read
Introduction to Object-Oriented Programming in Python
Object-Oriented Programming (OOP) is a programming paradigm centered around objects rather than functions and procedures. It represents a fundamental shift from procedural programming by organizing software design around data, or objects, rather than logic. In Python, OOP allows for the creation of reusable and modular code, which can be easily maintained and modified. The focus here is on creating classes that act as blueprints for objects, which encapsulate both data and behaviors.
The core concepts of OOP include classes, objects, inheritance, polymorphism, encapsulation, and abstraction. A class defines the structure and behavior (data and code) that the objects created from the class share. Objects are instances of classes that interact and collaborate with other objects to perform tasks. Inheritance allows new classes to inherit the properties and methods of existing ones, facilitating code reuse and the creation of hierarchical relationships. Polymorphism enables treating objects of different classes through a common interface. Encapsulation restricts direct access to some of an object’s components, which is a means of preventing unintended interference and misuse. Abstraction simplifies complex systems by modeling classes appropriate to the problem.
Python is a particularly popular language for implementing OOP due to its simplicity and readability. Its syntax is user-friendly, making it ideal for both beginners and experienced developers. Python also supports all the major OOP principles natively, allowing for a smooth and intuitive coding experience. Libraries like NumPy, Pandas, and Django further enrich its OOP capabilities by providing extensive support for mathematical, data processing, and web development applications, respectively.
Real-world applications of OOP in Python permeate various domains. In web development, frameworks like Django employ OOP to streamline the creation of complex web applications. Data analysis heavily relies on OOP principles in libraries such as Pandas, enabling efficient data manipulation and analysis. Additionally, in game development, engines like Pygame facilitate the design of interactive games using OOP to manage character behaviors and game states seamlessly.
Understanding Classes and Objects
In the realm of object-oriented programming (OOP), understanding the concepts of classes and objects is paramount. These components are the cornerstone of this programming paradigm, facilitating the efficient structuring and manipulation of data and functions. In Python, a class serves as a blueprint for creating objects (instances). It encapsulates data for the object and defines behaviors via methods.
A class is defined using the class
keyword followed by the class name. Inside the class, functions are defined to represent the behaviors, and variables to hold the data. Here's a basic example:
class Dog:
def __init__(self, name, age): self.name = name self.age = age def bark(self): print(f"{self.name} is barking.")# Creating an object of the Dog class
my_dog = Dog("Rex", 5)
print(my_dog.name) # Output: Rex
my_dog.bark() # Output: Rex is barking.
In the example above, the Dog
class is defined with an __init__
method, which is a special method called a constructor. It initializes the object's attributes—name
and age
. When the Dog
object my_dog
is created, these attributes are set with the values "Rex" and 5, respectively. The bark
method is a behavior the object can perform, demonstrated by the corresponding function printing a message.
Objects interact with each other through methods and attributes. Interactions between objects of different classes can model complex systems and behaviors. For example, a Dog
object could interact with a Park
object where multiple dogs are managed.
Classes also support inheritance and polymorphism, key principles in OOP to enhance reusability and flexibility. Inheritance allows a new class to inherit properties and behaviors of an existing class, whereas polymorphism lets different classes be treated through the same interface, ensuring that functions work with any class that implements the required interface.
Overall, classes and objects in Python enable a modular and organized approach to programming, fostering code readability and maintainability. Through practical usage and manipulation of these constructs, programmers can create robust and scalable applications.
Encapsulation in Python
Encapsulation is a cornerstone of object-oriented programming (OOP). It refers to the bundling of data and the methods that operate on that data within a single unit or class, while also restricting direct access to some of the object's components. This mechanism is crucial in maintaining the integrity and security of data, ensuring that it can only be modified in well-defined ways.
In Python, encapsulation is implemented using access specifiers, namely public, protected, and private. By default, all the members of a Python class are public, meaning they can be accessed from outside the class. However, using a single underscore prefix (e.g., _variable
), one can designate a member as protected; this is a convention indicating that the member should not be accessed directly outside the class, although it is still accessible. To make a member truly private, one can use a double underscore prefix (e.g., __variable
), which effectively restricts it from being accessed directly outside the class.
By implementing encapsulation, developers can control how data within an object is modified, thereby protecting the internal state of the object. For instance, consider a simple class BankAccount
where we encapsulate the balance:
This example demonstrates the use of private variables and public methods to access and modify the balance. By encapsulating the balance and providing controlled access through methods, the integrity of the balance is preserved, protecting it from improper changes.
Encapsulation not only promotes data security but also aids in maintaining modular, understandable code. It allows for the internal implementation of a class to change without affecting other parts of the program that rely on the class, facilitating easier maintenance and scalability.
```htmlPolymorphism in Python
Polymorphism is a foundational concept in object-oriented programming (OOP) that enables a single interface to represent different underlying forms (data types). In Python, polymorphism facilitates the implementation of methods that can work with objects of various classes, allowing for more flexible and scalable code development.
Polymorphism in Python is primarily achieved through method overriding and method overloading. Method overriding allows a subclass to provide a specific implementation of a method already defined in its superclass. This enables the subclass to modify or extend the behavior as needed. Consider the following example:
class Animal: def sound(self): return "Some sound"class Dog(Animal): def sound(self): return "Bark"class Cat(Animal): def sound(self): return "Meow"# Polymorphism in actionfor animal in (Dog(), Cat()): print(animal.sound())
In this example, both Dog
and Cat
classes override the sound
method of the Animal
superclass, providing their specific implementations. The loop demonstrates polymorphism by invoking the sound
method on each animal object, producing the correct output based on the object's class.
Method overloading, although less common in Python compared to other languages, involves defining multiple methods with the same name but different parameters within a single class. While Python does not natively support method overloading, similar behavior can be achieved using default parameters or variable-length arguments:
class Example: def add(self, a, b, c=0): return a + b + cex = Example()print(ex.add(2, 3)) # Output: 5print(ex.add(2, 3, 4)) # Output: 9
Here, the add
method can handle both two and three arguments, showcasing how Python can simulate method overloading.
The benefits of polymorphism in Python are numerous. It promotes code reusability, as the same interface can be employed by different classes. This capability is instrumental in designing extensible and maintainable software systems. By abstracting interactions with objects, polymorphism enables developers to build flexible software architectures, streamlining future enhancements and minimizing code redundancy.
```Abstraction in Python
Abstraction is one of the fundamental principles of Object-Oriented Programming (OOP) that simplifies complex systems by focusing on the essential features while hiding the unnecessary details. This is done by creating abstract classes and interfaces, thus enabling programmers to concentrate on high-level functionalities without delving into the implementation intricacies.
In Python, abstraction is achieved through the use of abstract classes. An abstract class can be considered a blueprint for other classes. It allows you to define methods that must be created within any child class built from the abstract class. Unlike a standard class, an abstract class cannot be instantiated directly. Instead, it lays down the contractual methods which need to be implemented by any subclass.
To implement abstract classes in Python, the abc
module is utilized, where ABC
stands for Abstract Base Class. A typical abstract class in Python looks something like this:
from abc import ABC, abstractmethodclass Shape(ABC): @abstractmethod def area(self): pass @abstractmethod def perimeter(self): pass
In this example, Shape
is an abstract class with two abstract methods: area
and perimeter
. Any subclass of Shape
must provide an implementation for these methods.
class Rectangle(Shape): def __init__(self, width, height): self.width = width self.height = height def area(self): return self.width * self.height def perimeter(self): return 2 * (self.width + self.height)
Here, the Rectangle
class inherits from the Shape
abstract class and provides concrete implementations for the area
and perimeter
methods. This demonstrates how abstraction allows for defining the structure without worrying about the details.
By using abstraction, developers can create more modular and maintainable code. It helps in reducing complexity, preventing redundancy, and promoting a clear separation of concerns. This abstraction principle, fundamental in Python programming, ensures a stable and flexible codebase, enabling easier enhancements and bug fixes.
```htmlInheritance in Python
Inheritance stands as a fundamental principle in object-oriented programming (OOP) that empowers new classes, termed as derived or child classes, to inherit attributes and methods from existing classes, known as base or parent classes. This essential feature fosters code reusability and contributes to a structured and modular application design. Understanding the various forms of inheritance is vital for leveraging its full potential in Python.
Single Inheritance occurs when a derived class inherits from just one base class.
class Parent: def func1(self): print("This is function one.") class Child(Parent): def func2(self): print("This is function two.") obj = Child() obj.func1() obj.func2()
By extending Parent, the Child class gains access to the attributes and methods of the Parent class.
Multiple Inheritance allows a child class to inherit from more than one parent class, offering broader functionality.
class Parent1: def func1(self): print("This is parent one.")class Parent2: def func2(self): print("This is parent two.")class Child(Parent1, Parent2): passobj = Child() obj.func1() obj.func2()
In this example, the Child class inherits methods from both Parent1 and Parent2.
Multilevel Inheritance includes inheritance from a derived class. A class derived from a derived class forms a multilevel inheritance relationship.
class Grandparent: def func1(self): print("This is the grandparent.")class Parent(Grandparent): def func2(self): print("This is the parent.")class Child(Parent): def func3(self): print("This is the child.")obj = Child() obj.func1() obj.func2() obj.func3()
The Child class shows multilevel inheritance by deriving from Parent, which in turn derives from Grandparent.
Hierarchical Inheritance entails multiple classes deriving from a single parent class.
class Parent: def func1(self): print("This is the parent.")class Child1(Parent): def func2(self): print("Child one derived from Parent.")class Child2(Parent): def func3(self): print("Child two derived from Parent.") obj1 = Child1() obj2 = Child2()obj1.func1() obj1.func2() obj2.func1() obj2.func3()
Here, both Child1 and Child2 inherit attributes and methods from Parent.
Hybrid Inheritance is a blend of two or more types of inheritance to achieve robust and flexible systems.
While inheritance significantly improves code reuse and organization, it demands careful consideration of when and how to use it. Overusing inheritance can lead to complex, rigid class hierarchies; thus, it is often beneficial to evaluate whether composition might be a simpler and more flexible choice. Understanding these principles ensures developers can effectively employ inheritance in Python programming.
```Company
Free Subscribe to us
©Copyright 2023 DATADRIX All Rights Reserved.
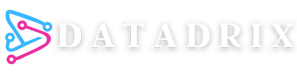
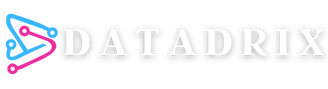
Courses
Location : Dilshad Garden, New Delhi
Contact Us : +91 - 9310936989